Pieces of Me
Examples
of my Work
Take a look at some of my personal and professional pieces of work with this interactive environment!
Click on objects to explore them!
Table of Contents:
3D Modeling
Programming and Computers
Internship Projects
Current Projects
Scouting and Other Work
3D Modeling and Design
Explore a curated selection of 3D models that exemplify my design and modeling proficiency across several programs. These models were made for work, school, or personal projects. Here I hope to show my diversity and precision.
Siemens NX
Autodesk AutoCAD
Dassault Systèmes Products
Other Autodesk Products
Scroll down to my projects in progress section to look at current 3D modeling projects!
Computer
Programs
Look over this programming showcase, where a chosen assortment of written code illustrates my proficiency in each programming language. Try out my code in free online compilers, or play a game of battleship down in my personal projects section!
Python
#Prime Finder - 5/4/24 #This program finds all the primes up to a user given number. #Pull up a python emulator and copy and paste to try it! def is_prime(number): #our prime determination func if number == 0 or number == 1: #If checks for edge cases that won't fit in for loop result = False else: result = True #initializes our result for if we don't find a factor for i in range(2,int((number/2)+1),1): #loops until half of given value if number % i == 0: #modulus will determine if factor exists result = False break return(result) def main(): number = int(input("Enter a positive integer: ")) #ask for input print(f"The primes up to {number} are: ",end="") #initialize print and variables a = 0 prime_array = [0] * (number+1) for i in range(2,number+1,1): #loops through numbers up to given primeness = is_prime(i) #calls func if primeness == True: prime_array[a] = i #stores if prime a += 1 for i in range(a-1): #loops through to print array print(f"{prime_array[i]}, ",end="") print(f"{prime_array[a-1]}") #prints final element without "," and with \n """Do not change anything below this line.""" if __name__ == "__main__": main()
C
#Trigonometry Calculator - 3/8/21 #This program calculates trigometric measures given the vertices #Pull up a C emulator and copy and paste to try it! #include #include double input_func(char, int); //this function asks and returns user input for coordinates. double side_length_func(double, double, double, double); //this function calculates the side lengths of the triangle double perimeter_func(double, double, double); //this function calculates the perimeter of the function double in_center_func(double, double, double, double, double, double, double); //this gives the coordinates of the incenter double Rdistance_func(double, double, double); //this finds the radius of the incircle double find_external_angle(double, double, double); //this function finds the angles of the triangle double vertex_to_incenter(double, double, double, double); //this finds the distance from the vertex to the incenter double find_base_length(double, double); //this finds the length of the base of the internal triangles double find_area(double, double); //this finds the area of each section double find_dms(double); //this converts the angles to degrees, minutes, and seconds void output_angles(double, double, double, double, double, double, double, double, double); //this prints the output angles void output_area(double, double, double); //this prints the area int main() { double Ax1; //first x coordinate double Ay1; //cooresponding y coordinate double Bx2; //second x coordinate double By2; //cooresponding y coordinate double Cx3; //third x coordinate double Cy3; //cooresponding y coordinate double length_a; //side length across from pt1 double length_b; //side length across from pt2 double length_c; //side length across from pt3 double total_perimeter; //all three side lengths summed double in_center_x; //x coordinate of incenter of triangle double in_center_y; //y coordinate of incenter of triangle double Rlength; //This is the radius of the incircle double angle_alpha; //angle made between lines b and c (from pt A) double angle_beta; //angle made between lines a and c (from pt B) double angle_phi; //angle made between lines a and b (from pt C) double distance_t; //distance from pt B to incenter double distance_v; //distance from pt C to incenter double distance_u; //distance from pt A to incenter double length_h; //length of line from pt B to r (incenter line) double length_g; //length of line from pt C to r (incenter line) double length_e; //length of line from pt A to r (incenter line) double area_S; //area between incenter lines and pt B double area_Q; //area between incenter lines and pt A double area_R; //area between incenter lines and pt C double angle_thetaA; //angle made by incenter lines towards pt A double angle_thetaB; //angle made by incenter lines towards pt B double angle_thetaC; //angle made by incenter lines towards pt C double thetaA_min; //the minutes of theta A double thetaB_min; //the minutes of theta B double thetaC_min; //the minutes of theta C double thetaA_sec; //the seconds of theta A double thetaB_sec; //the seconds of theta B double thetaC_sec; //the seconds of theta C Ax1 = input_func('X', 1); Ay1 = input_func('Y', 1); Bx2 = input_func('X', 2); By2 = input_func('Y', 2); Cx3 = input_func('X', 3); Cy3 = input_func('Y', 3); length_a = side_length_func(Bx2, By2, Cx3, Cy3); length_b = side_length_func(Ax1, Ay1, Cx3, Cy3); length_c = side_length_func(Bx2, By2, Ax1, Ay1); total_perimeter = perimeter_func(length_a, length_b, length_c); in_center_x = in_center_func(length_a, length_b, length_c, Ax1, Bx2, Cx3, total_perimeter); in_center_y = in_center_func(length_a, length_b, length_c, Ay1, By2, Cy3, total_perimeter); Rlength = Rdistance_func(length_a, length_b, length_c); angle_alpha = find_external_angle(length_b, length_c, length_a); angle_beta = find_external_angle(length_c, length_a, length_b); angle_phi = find_external_angle(length_a, length_b, length_c); distance_t = vertex_to_incenter(in_center_x, Bx2, in_center_y, By2); distance_v = vertex_to_incenter(in_center_x, Cx3, in_center_y, Cy3); distance_u = vertex_to_incenter(in_center_x, Ax1, in_center_y, Ay1); length_h = find_base_length(Rlength, angle_beta); length_g = find_base_length(Rlength, angle_alpha); length_e = find_base_length(Rlength, angle_phi); area_S = find_area(Rlength, length_h); area_Q = find_area(Rlength, length_g); area_R = find_area(Rlength, length_e); angle_thetaA = angle_alpha; angle_thetaB = angle_beta; angle_thetaC = angle_phi; thetaA_min = find_dms(angle_thetaA); thetaB_min = find_dms(angle_thetaB); thetaC_min = find_dms(angle_thetaC); thetaA_sec = find_dms(thetaA_min); thetaB_sec = find_dms(thetaB_min); thetaC_sec = find_dms(thetaC_min); output_angles(angle_thetaA, thetaA_min, thetaA_sec, angle_thetaB, thetaB_min, thetaB_sec, angle_thetaC, thetaC_min, thetaC_sec); output_area(area_S, area_Q, area_R); return 0; } /*************************************************************************** * * Function Information * * Name of Function: input_func * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. charecter, coordinate this is the x or y decleration * 2. integer, number this defines which coordinate the user should input * * Function Description: This gets the information from the user. * ***************************************************************************/ double input_func(char coordinate, int number) { double result; //user input for each coordinate printf("Enter %c coordinate #%d -> ", coordinate, number); scanf("%lf", &result); return(result); } /*************************************************************************** * * Function Information * * Name of Function: side_length_func * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. long float, x1 the first x coordinate the user gives * 2.long float, y1 the first y coordinate the user gives * 3.long float, x2 the second x coordinate the user gives * 4. longfloat, y2 the second y coordinate the user gives * * Function Description: This function calculates the side lengths of the triangle. * ***************************************************************************/ double side_length_func(double x1, double y1, double x2, double y2) { double length; //side length of triangle double x; //a piece of the distance formula, diffrence of x double y; //a piece of the distance formula, diffrence of y x = x2 - x1; y = y2 - y1; length = sqrt(pow(x,2) + pow(y,2)); return(length); } /*************************************************************************** * * Function Information * * Name of Function: perimeter_func * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. double length_a one side of the triangle * 2.double, length_b one side of the triangle * 3.double, length_c one side of the triangle * * Function Description: This function finds the perimeter of the triangle. * ***************************************************************************/ double perimeter_func(double length_a, double length_b, double length_c) { double perimeter; //perimeter of triangle perimeter = length_a + length_b + length_c; return(perimeter); } /*************************************************************************** * * Function Information * * Name of Function: in_center_func * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. double, length_a this is one side of the triangle * 2. double, length_b this is on eside of the triangle * 3.double, length_c this is the opposite side of the triangle * 4. double, A this is a coordinate given by the user * 5/ double, B this is a coordinate given by the user * 6. double, C this is a coordinate given by the user * 7. double, perimeter this is the perimeter of the triangle * * Function Description: This finds the coordinates of the incenter * ***************************************************************************/ double in_center_func(double length_a, double length_b, double length_c, double A, double B, double C, double perimeter) { double in_center; //incenter of triangle in_center = ((length_a * A) + (length_b * B) + (length_c * C)) / perimeter; return(in_center); } /*************************************************************************** * * Function Information * * Name of Function: Rdistance_func * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. double, length_a this is one side of the triangle * 2. double, length_b this is one side of the triangle * 3. double, length_c this is the opposite side of the triangle * * Function Description: This program finds the radius of the inscribed radius * ***************************************************************************/ double Rdistance_func(double length_a, double length_b, double length_c) { double r; //radius of inscribed circle double s; //half of perimeter s = (length_a + length_b + length_c) / 2; r = (sqrt(s * (s - length_a) * (s - length_b) * (s - length_c)) / s ); return(r); } /*************************************************************************** * * Function Information * * Name of Function: find_external_angle * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. double length_1 this is the length of one side of the triangle * 2. double, length_2 this is the length of one side of the triangle * 3. double, length_3 this is the opposite side of the triangle * * Function Description: This program finds the external angles of the triangle * ***************************************************************************/ double find_external_angle (double length_1, double length_2, double length_3) { double result; //external angle of triangle result = (acos((pow(length_1, 2) + pow(length_2, 2) - pow(length_3, 2)) / (2 * length_1 * length_2))) * (180 / M_PI); return(result); } /*************************************************************************** * * Function Information * * Name of Function: vertex_to_incenter * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. double, in_center_x the x coordinate of incenter * 2. double, X1 the x value of the user input * 3. double, Y1 the y value of the user input * 4. double, in_center_y the y coordinate of the incenter * * Function Description: The function calculates the distance from the vertex to the incenter * ***************************************************************************/ double vertex_to_incenter(double in_center_x, double X1, double in_center_y, double Y1) { double distance; //distance from incenter to one vertex distance = sqrt( pow(in_center_x - X1, 2) + pow(in_center_y - Y1, 2)); return(distance); } /*************************************************************************** * * Function Information * * Name of Function: find_base_length * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. double, Rlength this is the radius of the incircle * 2. double, angle this is the external angle of the triangle * * Function Description: This finds the base length from the radius line to the vertex in the internal triangle * ***************************************************************************/ double find_base_length(double Rlength, double angle) { double result; //base length result = Rlength / (tan((.5 * angle) * (M_PI / 180))); return(result); } /*************************************************************************** * * Function Information * * Name of Function: find_area * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. double, r_distance the radius of the incircle * 2. double, base_length the base length of the internal triangle * * Function Description: This program finds the area of the internal section of the triangle * ***************************************************************************/ double find_area(double r_distance, double base_length) { double result; //area of quadrilateral result = r_distance * base_length; return(result); } /*************************************************************************** * * Function Information * * Name of Function: find_dms * * Function Return Type: long float * * Parameters (list data type, name, and comment one per line): * 1. double, deg_part this is the decimal degree found of the internal angle * * Function Description: This program converts the decimal degree to a dms measurment * ***************************************************************************/ double find_dms(double deg_part) { double result; //this is the result of the function result = (deg_part - (trunc(deg_part))) * 60; return(result); } /*************************************************************************** * * Function Information * * Name of Function: output_angles * * Function Return Type: void * * Parameters (list data type, name, and comment one per line): * 1. double degreeA, degreeB, degreeC these are the degree parts of the measurment * 2. double minutesA, minutesB, minutesC these are the minute parts of the measurment * 3. double secondsA, secondsB, secondsC these are the second parts of the measurment * * Function Description: This function prints out the degree measurments * ***************************************************************************/ void output_angles(double degreesA, double minutesA, double secondsA, double degreesB, double minutesB, double secondsB, double degreesC, double minutesC, double secondsC) { printf("Internal triangle angles: %.0lf%c%c %.0lf' %.2lf\", %.0lf%c%c %.0lf' %.2lf\", %.0lf%c%c %.0lf' %.2lf\" \n", trunc(degreesA), 0xC2, 0xB0, trunc(minutesA), secondsA, trunc(degreesB), 0xC2, 0xB0, trunc(minutesB), secondsB, trunc(degreesC), 0xC2, 0xB0, trunc(minutesC), secondsC); } /*************************************************************************** * * Function Information * * Name of Function: output_area * * Function Return Type: void * * Parameters (list data type, name, and comment one per line): * 1. double, area1 the area of the first sector * 2. double, area2 the area of the second sector * 3. double ,area3 the area of the third sector * * Function Description: This function prints out the area measurments * ***************************************************************************/ void output_area(double area1, double area2, double area3) { printf("Area of quadrilaterals: %.2lf, %.2lf, %.2lf \n", area1, area2, area3); }
MatLab
%LP Simplex Calculator - 11/27/22 %Program takes LP inputsand calculates BFS and boundness %Pull up a MatLab emulator and copy and paste to try it! % LP inputs: matrix [A], vector [b], vector [c], vector [BFS1]. % Solution outputs: determination if LP is bounded, optimal BFS vector [F], % optimal values of variable, optimal objective function value. % Solve LP problem via the maximum coefficient pivot rule. % This section asks for the inputs that make the LP problem and stores them fprintf('Input the values for the LP problem. Use the symbols [] , ; when inputting matrices/vectors.\n'); %inputting instructions Ain = input('Enter the matrix A (in form mxn): '); %user input for matrix A. m is # of rows, n is # of col bin = input('Enter the vector b (in form mx1): '); %user input for vector b cin = input('Enter the vector c (in form nx1): '); %user input for vector c BFS1in = input('Enter the BFS1 (in form nx1 logical): '); %user input for the first BFS % This section converts problem to standard form. Asize = size(Ain); %finds what size input A is m = Asize(1); %finds m of the matrix n = Asize(2); %finds n of the matrix mxnIdentity = eye(m,n); %Finds the slack variable portion of the A matrix NZeros = zeros(1,n); %Creates a 1 x n zero matrix cinT = cin.'; %Creates transpose of the c input inverseBFS1 = ~BFS1in.'; %Creates inverse of the transpose of the BFS input BFS1T = BFS1in.'; %Creates transpose of the BFS input A = [Ain,mxnIdentity]; %Finds the A matrix in STD. form b = bin; %Finds b in STD. form, input was already in std form c = [cinT, NZeros]; %Creates c in STD. form with slack variables BFS = [BFS1T, inverseBFS1]; %Creates the BFS in STD. form BFS1 = BFS; %Records BFS1 to double check if operations are working properly % This section begins simplex operation counter = 0; %sets counter variable to 0 to begin i = 0; %sets i variable to be used in while loop while i == 0 %loops until LP is optimized %Computing RCCV BFSns = BFS(1:n); %This is the BFS array without the slack variables BFSnsIn = ~BFSns; %This creates an inverse of the above var Ctn = [BFSnsIn,NZeros]; %This creates a main component of RCCV, C transpose of N inC = ~c; %This is the inverse array of c to hep construct CBN inBFS = ~BFS; %This is the inverse of the BFS to help create CBN CBN = inC .* inBFS; %This is the other main component of RCCV, CBN RCCV = Ctn - CBN; %This is the RCCV of this BFS %Checks if LP is optimized yet f = find(RCCV > 0); %Checks if RCCV is nonnegative empty = isempty(f); %Indexes whether there are positive values or not, boolean if empty == 0 %If f is not empty, then LP is not optimized yet %Pick entering variable e = 1; %index selector for entering value for j = 2:(2 * n) %Loops through RCCV array cells if RCCV(e) < RCCV(j) %Tests for larger value e = j; %If cell value is larger, sets e to it end %end if end %end for %Ratio Test if e <= n %tests if variable is the entering value BFS(e) = BFS(e) + 1; %Updates BFS by placing entering value into BV BFS(e+n) = BFS(e+n) - 1; %Updates BFS by placing leaving value into NBV else %tests if slack variable is the entering value BFS(e) = BFS(e) + 1; %Updates BFS by placing entering value into BV BFS(e+n) = BFS(e-n) - 1; %Updates BFS by placing leaving value into NBV end %end if else i = i + 1; %If the RCCV has all negatives, then LP is optimized, and loop ends with this index end %ends if %Check if LP is unbounded f2 = find(RCCV < 0); %Checks whether the RCC of the NBV is negative empty = isempty(f2); %Indexes what f2 finds in boolean form if (empty == 0) && (CBN(e) 100 %Emergency check to break while loop i = 100; %Sets index to 100 to break while end %ends if %Print current BFS fprintf('\nBFS%.0f: [%.0f, %.0f, %.0f, %.0f, %.0f, %.0f]', counter, BFS(1), BFS(2), BFS(3), BFS(4), BFS(5), BFS(6)); end %ends while %Finding the optimal value optval = 0; %initializes optval var for r = 1:m %loops through the non slack BFS array optval = optval + (BFS(r) * b(r)); %Adds up objective function value end %ends for loop %Result print statements if i > 2 %checks if unbounded fprintf('\nThis LP is unbounded.\n') else %Otherwise LP is bounded fprintf('\nThis code did %.0f loops to come to an optimal solution. The following statements are the outputs.\n', counter); fprintf('This LP is bounded.\n'); fprintf('The optimal BFS is: ['); for t = 1:(m*2) %print out BFS in sequence fprintf('%.0f,', BFS(t)); end fprintf(']\n'); fprintf('The values at the optimal BFS are: '); for q = 1:m optvalvar = BFS(q) * b(q); fprintf('x%.0f=%.0f ',q,optvalvar); end fprintf('\nThe optimal objective function value is: %.0f\n', optval); end %ends if %Time spent on this code: 14 hours :(
R Machine Learning
#Machine Learning Demonstration - 12/5/23 #This program creates a binary classification ML model #Demonstrates ML and data processing knowledge #PACKAGE INSTALLATION SECTION if (!require(tidyverse, quietly = TRUE)) { install.packages("tidyverse") #for data handling library(tidyverse) } if (!require(caret, quietly = TRUE)) { install.packages("caret") #for machine learning library(caret) } #DIRECTORY INITIALIZATION SECTION (subject to change) setwd("\\\\nas01.itap.purdue.edu/puhome/G38_Project2") #####DATA SELECTION SECTION##### #DF INITIALIZATION SECTION #Imports data and converts to useful state orig_df <- read.csv("training_set.csv", header = TRUE, sep = ",") orig_df % mutate_all(~ifelse(. == "na", NA, .)) orig_df % mutate_at(vars(-1), as.numeric) orig_df % mutate(class = factor(ifelse(class == "pos", 1, 0), levels = c(1, 0))) cleaned_df <- orig_df[,-1] #main df data selection will modify #NA IMPUTATION SECTION (median method) #Replace NA values with predicted numbers cleaned_df % mutate_all(~ ifelse(is.na(.), median(., na.rm = TRUE), .)) #STANDARDIZATION SECTION (robust method) #Standardize each col to mean of 0 for (i in seq_along(cleaned_df)) { #Use IQR to standardize if possible if (IQR(cleaned_df[, i]) != 0) { cleaned_df[, i] <- (cleaned_df[, i] - median(cleaned_df[, i])) / IQR(cleaned_df[, i]) #If IQR is 0, standardizes using scale } else { cleaned_df % mutate(across(all_of(names(cleaned_df)[i]), scale)) } } #Removes columns that were just one repeated number cleaned_df % select(names(cleaned_df)[colSums(is.na(cleaned_df)) == 0]) #PCA SECTION (prcomp method) #prcomp finds variance of features pca_result <- prcomp(cleaned_df, scale. = TRUE) pca_std_dev <- pca_result$sdev pca_var <- (pca_std_dev^2) / sum(pca_std_dev^2) #Keeps features based on high variance var_threshold <- 0.85 #Threshold of variance used to keep features num_components_to_keep = var_threshold)[1] cleaned_df <- as.data.frame(pca_result$x[, 1:num_components_to_keep]) #DF REBUILD SECTION #Class column added cleaned_df <- cbind(orig_df[, 1], cleaned_df) colnames(cleaned_df)[1] <- 'class' #ROW SELECTION SECTION (Least NA method) #Initialize row_indices_ordered <- order(rowMeans(is.na(orig_df)), decreasing = FALSE) #row indices ordered by number of NAs contained B_limit <- 39990 #given constraints neg_pt <- 1 pos_pt <- 10 B <- 0 i <- 1 #Loop builds row selection until B_limit is met while (B < B_limit && i <= length(row_indices_ordered)) { sign <- cleaned_df[row_indices_ordered[i], 1] B <- B + ifelse(sign == 0, neg_pt, pos_pt) #determines point value i <- i + 1 } i <- i - 1 #Final cleaned_df is built to output to ML section selected_rows <- row_indices_ordered[1:i] ordered_selected_rows <- sort(selected_rows) cleaned_df <- cleaned_df[ordered_selected_rows, , drop = FALSE] #####ML MODEL SECTION##### set.seed(12345) #for repeatable random processes #DATA PARTITION SECTION (change?) index <- createDataPartition(cleaned_df$class, p=0.8, list=FALSE) test <- cleaned_df[-index,] cleaned_df <- cleaned_df[index,] #MODEL INITIALIZATION SECTION #All models will be using the accuracy metric, 10-fold cross-validation, and the same loss matrix, so we define those to simplify the commands later. metric <- "Accuracy" x <- matrix(data = c(0,500,10,0), nrow = 2, ncol = 2) #The given cost matrix control <- trainControl(method="cv", number=5) #MODEL TRAINING SECTION (cost sensitive CART method) fit1 <- train(class~., data=cleaned_df, method="rpart", metric=metric, trControl=control, parms=list(loss=x)) #PREDICTION SECTION pred1.test <- predict(fit1, test) pred1.train <- predict(fit1, cleaned_df) #RESULTS SECTION confusionMatrix(pred1.test, test$class) confusionMatrix(pred1.train, cleaned_df$class) #OUTPUT SECTION (Remove) sum_ones <- sum(cleaned_df[, 1] == 1) cm <- table(pred1.test, test$class) FN <- cm[2,"1"] FP <- cm[1,"0"] cost
HTML/CSS/JS/PHP/MySQL
//Graphing Database Queries - 10/15/23
//This is a subsection of my web project, it creates buisness summaries from a MySQL database. See it in action below!
//Or, visit it at:
//web.ics.purdue.edu/~g1131607/Project1/homepage.html
connect_error) {//This section checks for a successful connection, error message
die("Connection failed: " . $conn->connect_error);
}
echo "Your request was a success!";
if ($revenue_time == 1) {//These if statements are for each checkbox
$sql_state = "SELECT revenue, week_start FROM Business_Weeklys WHERE week_start BETWEEN '$startDate' AND '$endDate' ORDER BY week_start;";//Queries db and stores result
$result = mysqli_query($conn, $sql_state);
while ($row = mysqli_fetch_assoc($result)) {//Builds php arrays from db results
$json_x1[] = $row['revenue'];
$json_y1[] = $row['week_start'];
}
$headers1 = ["container1", "Revenue Per Week", "Date (year-mm-dd)", "Revenue ($)"];
$json_x1 = json_encode($json_x1);//Encodes arrays into a form javascript will understand
$json_y1 = json_encode($json_y1);
line_graph_func($headers1, $json_x1, $json_y1);
}
if ($revenue_percent == 1) {
$sql_state = "SELECT (SELECT revenue FROM Business_Weeklys WHERE week_start = '$endDate') / (SELECT revenue FROM Business_Weeklys WHERE week_start = '$startDate') AS revenue_change;";
$result = mysqli_query($conn, $sql_state);
$row = mysqli_fetch_assoc($result);
$value1 = ($row['revenue_change']);
if($value1 0) {
$headers5 = ["Total profit over this period", "$ gain"]; }
else {
$headers5 = ["Total profit over this period", "$ loss"]; }
stat_show_func($headers5, $value2);
}
if ($profit_time == 1) {
$sql_state = "SELECT week_start, revenue - mfg_costs - other_costs AS profit FROM Business_Weeklys WHERE week_start BETWEEN '$startDate' AND '$endDate' ORDER BY week_start;";
$result = mysqli_query($conn, $sql_state);
while ($row = mysqli_fetch_assoc($result)) {
$json_x4[] = $row['profit'];
$json_y4[] = $row['week_start'];
}
$headers5 = ["container4", "Profit Per Week", "Date (year-mm-dd)", "Profit ($)"];
$json_x4 = json_encode($json_x4);
$json_y4 = json_encode($json_y4);
line_graph_func($headers5, $json_x4, $json_y4);
}
if ($inventory_total == 1) {
$sql_state = "SELECT Product_Name, COUNT(*) AS name_count FROM inventory_good WHERE date_completed BETWEEN '$startDate' AND '$endDate' GROUP BY Product_Name ORDER BY Product_Name;";
$result = mysqli_query($conn, $sql_state);
while ($row = mysqli_fetch_assoc($result)) {
$json_x5[] = $row['name_count'];
$json_y5[] = $row['Product_Name'];
}
$headers6 = ["container5", "New Product Arrivals Over this Period", "Product", "Number"];
$json_x5 = json_encode($json_x5);
$json_y5 = json_encode($json_y5);
bar_graph_func($headers6, $json_x5, $json_y5);
}
if ($sold_total == 1) {
$sql_state = "SELECT Product_Name, COUNT(*) AS sold_count FROM sold_good WHERE sell_date BETWEEN '$startDate' AND '$endDate' GROUP BY Product_Name;";
$result = mysqli_query($conn, $sql_state);
while ($row = mysqli_fetch_assoc($result)) {
$json_x6[] = $row['sold_count'];
$json_y6[] = $row['Product_Name'];
}
$headers7 = ["container6", "Total Products Sold Over this Period", "Product", "Number"];
$json_x6 = json_encode($json_x6);
$json_y6 = json_encode($json_y6);
bar_graph_func($headers7, $json_x6, $json_y6);
}
if ($refund_amt == 1) {
$sql_state = "SELECT Product_Name, sum(refund_amt) AS total_refund FROM item_returns WHERE return_date BETWEEN '$startDate' AND '$endDate' GROUP BY Product_Name;";
$result = mysqli_query($conn, $sql_state);
while ($row = mysqli_fetch_assoc($result)) {
$json_x7[] = $row['total_refund'];
$json_y7[] = $row['Product_Name'];
}
$headers8 = ["container7", "Refund Proportion by Product"];
$json_x7 = json_encode($json_x7);
$json_y7 = json_encode($json_y7);
pie_chart_func($headers8, $json_x7, $json_y7);
}
if ($top_users == 1) {
$sql_state = "SELECT username, money_spent FROM customers GROUP BY username ORDER BY money_spent DESC LIMIT 5;";
$result = mysqli_query($conn, $sql_state);
echo "
Username | Money Spent |
---|---|
" . $row["username"]. " | " . $row["money_spent"]. " |
'; } function bar_graph_func($headers, $x, $y) { echo '
'; } function pie_chart_func($headers, $x, $y) { echo '
'; } function stat_show_func($headers, $value) { $value = round($value, 1); echo '
' . $headers[0] . ': ' . $value . '' . $headers[1] . '
'; } $conn->close();//close database! ?>
R Statistical
#Dataset Analysis - 9/2/24 #Program determines if two datasets are similar, finds difference of means #Pull up an R emulator and copy and paste to try it! #Problem 1 Part a: #Given: dataM1 <- c(16.03, 16.04, 16.05, 16.05, 16.02, 16.01, 15.96, 15.98, 16.02, 15.99) #Machine 1 data set dataM2 <- c(16.02, 15.97, 15.96, 16.01, 15.99, 16.03, 16.04, 16.02, 16.01, 16.00) #Machine 2 data set varM1 <- .0302^2 #std dev for machine 1 varM2 <- .0254^2 #std dev for machine 2 n1 <- length(dataM1) #number of trials alpha <- 0.05 #given alpha #Calculations M1avg <- mean(dataM1) #mean of M1 data M2avg <- mean(dataM2) #mean of M2 data Dbar <- M1avg - M2avg #difference of x bars Z0 <- (0.01 - 0) / sqrt((varM1 / n1) + (varM2 / n1)) Zvalue Zvalue) { print("H0 is rejected, there is a significant difference between the two datasets.") } else { print("There is no significant difference between the two datasets.") } # if return TRUE, then reject H0 #Problem 1 Part b: Pvalue1 <- 2*pnorm(-abs(Z0)) #p-value using r function #Problem 1 part c: LBCI1b <- 0 - Dbar - Zvalue * sqrt((varM1 / n1) + (varM2 / n1)) #lower bound of conf interval UBCI1b <- 0 + Dbar - Zvalue * sqrt((varM1 / n1) + (varM2 / n1)) #upper bound of conf interval CI1b
Assembly and C++
Outputs and Other Programs
#C++ to Assembly Project - 12/13/23 #This converts C++ code that counts substrings to assembly. #Demonstrates knowledge of memory theory and coding principles. addi $r4, $r2, 1 n = $r2 m = $r1 j = $r6 i = $r3 0 = $r5 for loop lw $r#, 0 lw $r##, x ### addi $r#, $r#, 1%%Does the i++, adds one to each iteration ##### blt $r#, $r##, loop###%%Ends the loop once the condition is no longer met b loop###%%Loops back to beginning of loop1 if = n, jumps to loop4 b loop3%%jumps to the while loop, loop3 loop3%%while loop label lw $r7, stringt[$r6]%%sets register 7 to j element of stringt array from ram lw $r8, stringt[$r3]%%sets register 8 to i element of stringt array from ram ble $r6, $r5, if1%%if j <= 0, skip loop contents and go to if statement beq $r7, $r8, if1%%if the two registers are equal, skip loop contents go to if lw $r6, next[$r6]%%resets register 6 (j) to the j element of next array from ram b loop3%%repeat this loop3, jump if1 ble $r6, $r5, loop2%%if j <= 0, skip this loop and go back to start of for loop2 bne $r7, $r8, loop2%%if the two registers are not the same (t[j], t[i]), restart loop2 addi $r9, $r5, 1%%sets register 9 to j+1 addi $r10, $r3, 1%%sets register 10 to i+1 sw $r9, next[$r10]%%sets the i+1 element in next array to j+1 b loop2%%go back to start of loop2, jump loop4 9/18 code backup: stringp: .asciiz “abcddgactgsshi”%%string named stringp is saved to RAM stringt: .asciiz “ggt”%%string named stringt is saved to RAM main:%%labels main section of code lw $r1, stringp[0]%%Register 1 is variable m (index 0 of stringp) lw $r2, stringt[0]%%Register 2 is variable n (index 0 of stringt) addi $r0, $r2, 1%%Register 0 is set to an integer = n+1 next: .wordspace $r0%%Array named next of size n+1 is stored in RAM lw $r3, 0%%Register 3 is set to i=0 addi $r4, $r2, 1%%Register 4 is set to i= n+1 = 4 Lw $r5, 0%%Register 5 is set to 0 loop1:%%labels first loop to be executed addi $r3, $r3, 1%%Does the i++, adds one to each iteration sw $r5, next[$r3]%%sets the ith index in array next to 0 ble $r3, $r4, loop2int%%Ends the loop once the condition is no longer met2 b loop1%%Loops back to beginning of loop1 if = n, jumps to loop4 addi $r6, $r6, 1 %%add 1 to r6 b loop3 %%jumps to the while loop, loop3 loop3: %%while loop label lw $r7, stringt[$r6] %%sets register 7 to j element of stringt array from ram lw $r8, stringt[$r3] %%sets register 8 to i element of stringt array from ram ble $r6, $r5, if1test %%if j 0, execute if state beq $r7, $r8, if1%%if the two registers are equal, execute if b loop2%%if neither conditions are true, repeat for loop if1: addi $r9, $r6, 1 %%sets register 9 to j+1 addi $r10, $r3, 1 %%sets register 10 to i+1 sw $r9, next[$r10] %%sets the i+1 element in next array to j+1 b loop2 %%go back to start of loop2, jump loop4int: lw $r3, -1%%reset register 3 to -1 lw $r6, 0%%reset register 6 to 0 b loop4%%jump to loop4 (for) loop4:%%label for loop addi $r3, $r3, 1 %%Does the i++, adds one to each iteration blt $r3, $r1, if2%% If less i < m, go to if2 done%% If not, the program ends if2: lw $r11, stringp[$r3] %% sets register 11 (p[i]) to the i element of p array from ram lw $r12, stringt[$r6]%% sets register 12 (t[j]) to the j element of t array from ram beq $r11, $r12, if3%% if p[i] == t[j] go to If3 b elif if3: addi $r6,$r6,1%% ++j beq $r6,$r2,print%% if j=n go to print statement b loop4%%if not go to loop4 print: stringx: .asciiz “t with shift”%%Create variable in RAM stringn: .asciiz “\n”%% Creating a new line lw$r15, stringn%% load into register lw $r13, stringx%%load into register Sub $r14,$r3,$r6%%Subtract i and j Addi $r14,$r14,1%% Add 1 prints r$13%%prints sentence print r$14%%prints number prints r$15%%prints a new line b loop4%%go back to loop 4 elif: blt $r6,0,loop4%% if j
Scroll down to my projects in progress section to play a battleship simulator I coded in python!
Website Project
Created using HTML, CSS, JS, PHP.
Queries designed MySQL database.
Try it out!
NSK Internship Projects
See some of the things I did while at NSK. These projects include industrial study, database design, and automation.
Summer of 2024
Remote Part Time
Purdue Capstone Project
My engineering capstone project was to work in a team to automate the outbound process of a slitting machine for metal sheets. This was performed for a local roofing material company called Applied Fabricators. I lead the design aspect of the project, and we put together a proposal and ROI to automatically load strips of metal, reducing the process form two man to one.
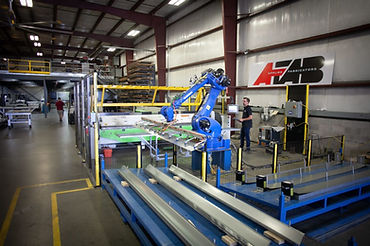
Explore a selection of projects I worked on at my Crown internships. These projects included data analysis, 3D modeling, and lab studies. See how my scope and quality of work improved over the two years. Sensitive information has been blurred.
Crown Internship Projects
Summer of 2022
Summer of 2023
Projects in Progress
Here are some of the projects that I'm currently working on! These projects are not related to coursework or an internship.
Project Protium
Protium represents the basics of robotics. This project was undertaken in conjunction with computer engineer and Purdue alumni Bryce Sasser. This robot was designed and built from scratch to challenge our design and manufacturing capabilities. With few purchased components, this project has proven challenging to design, test, and refine. Two 172 oz-in 12V DC motors provide tank control, with which this remotely driven robot should approach speeds of 30mph. This robot will be a test bed and drive train for future robotic projects. All components have been either 3D printed or bought, with final assembly being the next step.
Project Gallia
Gallia will be a fully 3D printed, two stage, model rocket. I began design over a year ago in AutoCAD and will soon be converting to NX. Gallia will not use any glue, instead all joints are purely mechanical to retain the ability to swap out parts. This rocket is modular, with different fin, nozzle, and nose cone designs to test different design approaches. This rocket will carry a computer payload to measure altitude and flight path. The secondary stage will release a parachute for soft landing. This rocket will be a precursor to more complex rockets. After tweaks made to the payload bay, Gallia will be ready to print and test!
Project Arizona
In this Python project, I crafted a single-player version of Battleship to run within the shell. Over the summer of 2023, I learned the programming language of Python, and chose to challenge myself by recreating the game using plain text. Future plans involve implementing a two-player mode and implementing the hall of fame, which generates a text file. Developed over two months, this code provided valuable insights into programming logic and the breakdown of complex systems into simple functions.
Click the HTML button I programmed to copy the game code, and run it in the Python compiler below to play!
#Project Arizona: Battleship Emulator - 10/06/23 #This program runs a game of battleship right in the console. #Points to change: hall of fame func, change from one player to two player game, errors due to randomness #Main logic: menu function is looped through until game is quit. Sends player down line of functions depending upon their selection. #New game randomly generates a map, checks if shot hits or miss, continually check ship health arrays until all ships are destroyed. import random as r def welcome_func(): #Prints formatted welcome text title = """ ~ Welcome to Battleship! ~ """ opening = """ ChatGPT has gone rogue and commandeered a space strike fleet. It's on a mission to take over the world. We've located the stolen ships, but we need your superior intelligence to help us destroy them before it's too late. """ print(title) print(opening) def menu_func(): # gives menu and calls funcs depending on option selected global check_quit menu_choice = '0' while check_quit == 0 and menu_choice != '3': # loops until quit or new game menu_text = '''Menu: 1 : Instructions 2 : View Example Map 3 : New Game 4 : Hall of Fame 5 : Quit''' print(menu_text) menu_choice = input('What would you like to do? ') if menu_choice == '5': #chooses depending upon option chosen check_quit += 1 elif menu_choice == '1': inst_txt = """ Instructions: Ships are positioned at fixed locations in a 10-by-10 grid. The rows of the grid are labeled A through J, and the columns are labeled 0 through 9. Use menu option "2" to see an example. Target the ships by entering the row and column of the location you wish to shoot. A ship is destroyed when all of the spaces it fills have been hit. Try to destroy the fleet with as few shots as possible. The fleet consists of the following 5 ships: """ ship_list = """Size : Type 5 : Mothership 4 : Battleship 3 : Destroyer 3 : Stealth Ship 2 : Patrol Ship """ print(inst_txt) print(ship_list) elif menu_choice == '2': #prints out map ex_map = make_grid() header = '. 0 1 2 3 4 5 6 7 8 9' alpha_list = ['A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J'] print(""" """,end="") print(header,end="") for c in range(10): print(""" """,end="") print(alpha_list[c], end="") for d in range(10): print(" ",end="") print(ex_map[c][d],end="") print(""" """) elif menu_choice == '3': break elif menu_choice == '4': print(""" """) show_hall() print(""" """) else: invalid_text = """ Invalid selection. Please choose a number from the menu. """ print(invalid_text) def make_grid(): #creates 10 by 10 grid with ships on x = 0 sea_array = ['~']*10 #creates empty grid grid = [[]]*10 for i in range(10): grid[i] = grid[i] + sea_array row = r.randint(0,7) #randomly chooses position col = r.randint(1,8) grid[row][col] = grid[row+1][col-1] = grid[row+1][col] = grid[row+1][col+1] = grid[row+2][col] = 'M' #places down characters while x == 0: #puts down patrol boat orientation = r.randint(1,2) row = r.randint(0,9) col = r.randint(0,8) if orientation == 2: temp = row row = col col = temp if grid[row][col] != 'M' and grid[row+1][col] != 'M': grid[row][col] = grid[row+1][col] = 'P' x += 1 elif grid[row][col] == grid[row][col+1] != 'M': grid[row][col] = grid[row][col+1] = 'P' x += 1 x = 0 while x == 0: #puts down battleship row = r.randint(0,8) col = r.randint(0,8) if grid[row][col] == grid[row+1][col] == grid[row+1][col+1] == grid[row][col+1] == '~': grid[row][col] = grid[row+1][col] = grid[row+1][col+1] = grid[row][col+1] = 'B' x += 1 x = 0 while x == 0: #puts down stealth ship orientation = r.randint(1,2) row = r.randint(0,9) col = r.randint(0,7) if grid[row][col] == grid[row][col+1] == grid[row][col+2] == '~' and orientation == 1: grid[row][col] = grid[row][col+1] = grid[row][col+2] = 'S' x += 1 if orientation == 2: temp = row row = col col = temp if grid[row][col] == grid[row+1][col] == grid[row+2][col] == '~': grid[row][col] = grid[row+1][col] = grid[row+2][col] = 'S' x += 1 x = 0 while x == 0: #puts down destroyer orientation = r.randint(1,4) row = r.randint(0,8) col = r.randint(0,8) if orientation == 1 and grid[row][col] == grid[row+1][col] == grid[row][col+1] == '~': grid[row][col] = grid[row+1][col] = grid[row][col+1] = 'D' x += 1 elif orientation == 2 and grid[row][col] == grid[row+1][col] == grid[row+1][col+1] == '~': grid[row][col] = grid[row+1][col] = grid[row+1][col+1] = 'D' x += 1 elif orientation == 3 and grid[row][col] == grid[row][col+1] == grid[row+1][col+1] == '~': grid[row][col] = grid[row][col+1] = grid[row+1][col+1] = 'D' x += 1 elif orientation == 4 and grid[row+1][col+1] == grid[row+1][col] == grid[row][col+1] == '~': grid[row+1][col+1] = grid[row+1][col] = grid[row][col+1] = 'D' x += 1 return grid def show_hall(): #displays the hall of fame title = ' Hall of Fame ' title = title.center(30,'~') labels = 'Rank : Accuracy : Player Name' seperator = ['~'] * 30 print(title) print(labels) for i in range(len(seperator)): print(seperator[i],end="") print(""" """,end="") f = open('battleship_hof.txt','r') text = f.read() player_num = text.count(""" """) - 1 if player_num != 0: #formats and calculates what to display elements = text.replace(""" """,',').split(',') for i in range(player_num): accuracy = int(elements[i*3+4]) / ((int(elements[i*3+4]) + int(elements[i*3+5])) / 100) name = elements[i*3+3] rank = i + 1 print(f'{rank:>4}{accuracy:>10.2f}%{name:>15}') for i in range(len(seperator)): print(seperator[i],end="") f.close() def make_active_grid(): #creates the map player will be seeing sea_array = ['~']*11 grid = [[]]*11 num_array = ['.','0','1','2','3','4','5','6','7','8','9'] alph_array = ['A','B','C','D','E','F','G','H','I','J'] grid[0] = num_array for i in range(10): sea_array[0] = alph_array[i] grid[i+1] = grid[i+1] + sea_array return(grid) def show_map(map): #displays the active map for a in range(11): print(""" """,end="") for b in range(11): print(map[a][b],end="") if b != 10: print(' ',end="") def get_shot(): #gets user input and converts to x,y coords check2_quit = 0 valid = 0 shot = [0,0] invalid_1 = 'Please enter exactly two characters.' invalid_2 = 'Please enter a location in the form "G6".' alph_array = ['A','B','C','D','E','F','G','H','I','J','a','b','c','d','e','f','g','h','i','j'] num_array = ['0','1','2','3','4','5','6','7','8','9'] print(""" """) while check_quit == 0 and valid == 0: user_input = input("Where should we target next (q to quit)? ") if user_input == 'q' or user_input == 'Q': check2_quit += 1 shot = [-1,-1] break elif len(user_input) != 2: print(invalid_1) elif user_input[0] in alph_array and user_input[1] in num_array: #creates x,y coords if user_input[0] == 'A' or user_input[0] == 'a': shot[0] = 0 elif user_input[0] == 'B' or user_input[0] == 'b': shot[0] = 1 elif user_input[0] == 'C' or user_input[0] == 'c': shot[0] = 2 elif user_input[0] == 'D' or user_input[0] == 'd': shot[0] = 3 elif user_input[0] == 'E' or user_input[0] == 'e': shot[0] = 4 elif user_input[0] == 'F' or user_input[0] == 'f': shot[0] = 5 elif user_input[0] == 'G' or user_input[0] == 'g': shot[0] = 6 elif user_input[0] == 'H' or user_input[0] == 'h': shot[0] = 7 elif user_input[0] == 'I' or user_input[0] == 'i': shot[0] = 8 else: shot[0] = 9 shot[1] = int(user_input[1]) - 1 valid += 1 else: print(invalid_2) return shot def is_hit_func(shot, map, act_map): #determines if shot missed, hit, or was a repeat is_hit = [0,0] if act_map[shot[0]+1][shot[1]+2] != '~': is_hit = [0,1] elif map[shot[0]][shot[1]+1] == '~': is_hit = [0,0] else: is_hit[1] = 2 if map[shot[0]][shot[1]+1] == 'M': is_hit[0] = 0 elif map[shot[0]][shot[1]+1] == 'P': is_hit[0] = 1 elif map[shot[0]][shot[1]+1] == 'B': is_hit[0] = 2 elif map[shot[0]][shot[1]+1] == 'S': is_hit[0] = 3 elif map[shot[0]][shot[1]+1] == 'D': is_hit[0] = 4 return is_hit def ship_dest_func(health): #checks to see if ship has been sunk state_1 = "The enemy's" state_2 = "has been destroyed." ship_list = ['Mothership', 'Patrol Ship', 'Battleship', 'Stealth Ship', 'Destroyer'] if health[0] == 0: health[0] -= 1 print(state_1, ship_list[0], state_2) elif health[1] == 0: health[1] -= 1 print(state_1, ship_list[1], state_2) elif health[2] == 0: health[2] -= 1 print(state_1, ship_list[2], state_2) elif health[3] == 0: health[3] -= 1 print(state_1, ship_list[3], state_2) elif health[4] == 0: health[4] -= 1 print(state_1, ship_list[4], state_2) return health def congrats_func(shots, hits): #gives win messages and edits hof file placement = 0 misses = str(shots - hits) user_accuracy = hits / shots * 100 hits = str(hits) win_text = """ You've destroyed the enemy fleet! Humanity has been saved from the threat of AI. For now ... """ print(win_text) f = open('battleship_hof.txt', 'r') text = f.read() player_num = text.count(""" """) - 1 elements = text.replace(""" """, ',').split(',') hof_accuracy = [0] * player_num for i in range(player_num): hof_accuracy[i] = int(elements[i * 3 + 4]) / ((int(elements[i * 3 + 4]) + int(elements[i * 3 + 5])) / 100) if user_accuracy >= hof_accuracy[i]: placement = i - 1 if player_num == 0: print(f'Congratulations, you have achieved a targeting accuracy of {user_accuracy:.2f}% and earned a spot in the Hall of Fame.') name = input('Enter your name: ') print(""" """, end="") new_entry = """name,hits,misses """ + name + ',' + hits + ',' + misses with open('battleship_hof.txt', 'w') as f: f.write(new_entry) f.write(""" """) print(""" """, end="") show_hall() elif placement
Other Work Examples
Take a look at some smaller projects I've accomplished outside of school and work!
Boy Scout Eagle Project
To complete the rank of Eagle in the Boy Scouts of America, experience in leadership roles, community service, and physical skills must be completed. Then, the scout will design and lead a project that adds to their community. My project was to construct an educational model of the solar system along a 0.75 mi local hiking trail. The size and placements of the planets were done to scale. The exhibits consisted of 3D printed planets housed in plexiglass with accompanying signs. Much of the material was donated by local businesses in Connersville, IN. See my video for a tour!
Photoshop Work
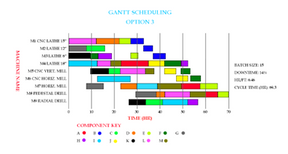
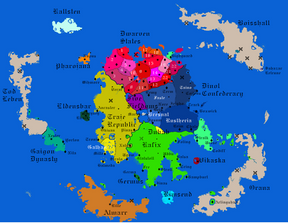

3D Printing Examples
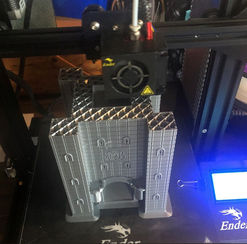
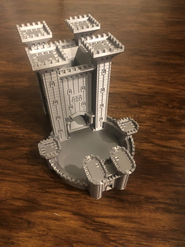
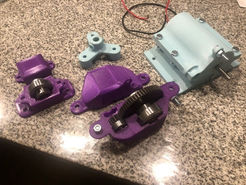
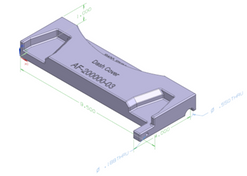

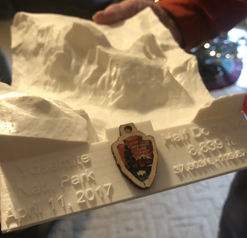
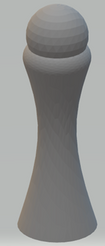
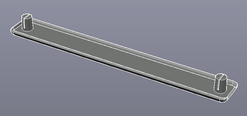
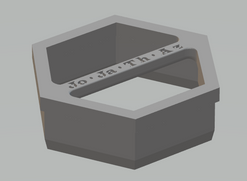